POC: OpenGLES with Orientation Sensor
This is a proof-of-concept (P.O.C.) for rendering OpenGL world by using the phone's Orientation Sensor as an input.
First I'll draw a crate in 3D space, then I'll set the camera (View Point) to look at it. whenever a user look away (roll/pitch/yaw) the crate, it will disappear from screen until the user point the phone back to that direction.
here's an illustration of what I mean:
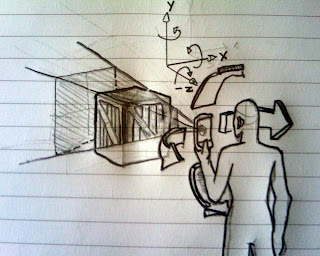
code segments:
Here's some screen shots of this P.O.C.:


Regards,
Avatar Ng
First I'll draw a crate in 3D space, then I'll set the camera (View Point) to look at it. whenever a user look away (roll/pitch/yaw) the crate, it will disappear from screen until the user point the phone back to that direction.
here's an illustration of what I mean:
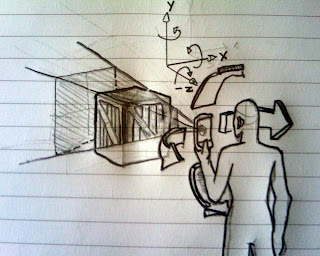
code segments:
public class GLSurfaceViewActivity extends Activity implements SensorEventListener{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// ...
// --- init sensor ---
mSensorManager = (SensorManager)getSystemService(SENSOR_SERVICE);
sensorList = mSensorManager.getSensorList(Sensor.TYPE_ORIENTATION);
orientationSensor = sensorList.get(0);
// ...
mSensorManager.registerListener(this,
orientationSensor,SensorManager.SENSOR_DELAY_FASTEST);
}
/**
*
**/
public void onSensorChanged(SensorEvent event){
if (event.sensor == orientationSensor) {
orientationValues = event.values;
// values[0]:
// Azimuth, angle between the magnetic north direction and the Y axis,
// around the Z axis (0 to 359). 0=North, 90=East, 180=South, 270=West
float rz = event.values [0];
// values[1]:
// Pitch, rotation around X axis (-180 to 180),
// with positive values when the z-axis moves toward the y-axis.
float rx = event.values [1];
// values[2]:
// Roll, rotation around Y axis (-90 to 90),
// with positive values when the x-axis moves away from the z-axis.
float ry = -event.values [2];
// pass these sensor values into renderer ...
}
}
}
public class CrateRenderer implements GLSurfaceView.Renderer {
// ...
public void onDrawFrame(GL10 gl) {
// rotate the camera around object
gl.glRotatef(rx, 1, 0, 0);
gl.glRotatef(ry, 0, 1, 0);
gl.glRotatef(rz, 0, 0, 1);
// ...
mCube.draw(gl, textureFilter);
}
// ...
}
Here's some screen shots of this P.O.C.:




Regards,
Avatar Ng
Comments
Post a Comment